API pagination becomes crucial to manage data efficiently and provide a seamless user experience. In this blog, we'll explore the concept of API pagination, its importance, best practices, and strategies for effective implementation.
What is API Pagination?
API pagination refers to the method of dividing a large set of data into smaller, manageable chunks or "pages" to be retrieved sequentially. This technique is essential for APIs that handle large datasets, as it prevents overwhelming the client and server with excessively large responses and enhances performance.
When a client makes a request to an API that supports pagination, the response typically includes only a subset of the total data. The client then uses information provided by the API to fetch additional pages as needed. This approach helps in optimizing the use of resources, improving response times, and ensuring a better user experience.
Using embedded integration, Klamp automates its apps in 3 days. Book a meeting now.
Why is API Pagination Important?
API pagination is crucial for several reasons:
Optimization: Large datasets can lead to slow response times and increased server load. Pagination helps in breaking down the data into smaller, more manageable pieces, which can significantly improve performance.
Reduced Network Traffic: By sending smaller chunks of data, pagination reduces the amount of data transmitted over the network. This is particularly important for mobile devices and users with limited bandwidth.
User Experience: Pagination enhances the user experience by providing data in a more digestible format. Users can navigate through pages of data rather than waiting for a massive dataset to load all at once.
Error Handling: Smaller, paginated responses make it easier to handle errors and retry failed requests without needing to reprocess the entire dataset.
Common Pagination Strategies
There are several common strategies for implementing API pagination. Each method has its pros and cons, and the choice of strategy depends on the specific use case and requirements.
1. Offset-Based Pagination
Offset-based pagination involves specifying an offset (or starting point) and a limit (number of items per page). For example, a request might specify an offset of 20 and a limit of 10, which would return items 21 through 30.
Pros:
- Simple to implement and understand.
- Easy to manage pagination in client-side code.
Cons:
- Performance can degrade with large datasets as offsets increase, causing slower query times.
- Items may be missed or duplicated if the dataset changes between requests.

2. Cursor-Based Pagination
Cursor-based pagination uses a unique identifier (cursor) to mark the position in the dataset. Each response includes a cursor for the next page. This method is more efficient for large datasets and provides more consistent results.
Pros:
- More efficient for large datasets and avoids performance issues with high offsets.
- Consistent results even if the dataset changes between requests.
Cons:
- More complex to implement compared to offset-based pagination.
- Requires handling of cursors and state management.

3. Keyset Pagination
Keyset pagination is cursor-based but uses a specific column value (key) to determine the next page. This method is effective when dealing with sorted data.
Pros:
- Efficient and avoids performance issues with large offsets.
- Suitable for datasets that are ordered by a specific field.
Cons:
- Requires the dataset to be ordered by the key used for pagination.
- May not be suitable for all types of datasets.
Best Practices for API Pagination
Implementing API pagination effectively requires adhering to best practices to ensure optimal performance and user experience. Here are some key practices to follow:
1. Choose the Right Pagination Strategy
Select the pagination strategy that best fits your use case. Offset-based pagination is simple and effective for smaller datasets, while cursor-based and keyset pagination are better suited for larger datasets and more dynamic environments.
2. Provide Clear Metadata
Include clear metadata in the API response to help clients navigate the paginated data. Common metadata includes:
- ‘total_count’: Total number of items available.
- ‘current_page’: The current page number or offset.
- ‘total_pages’: Total number of pages available.
- ‘next_page’: URL or cursor for the next page.
3. Implement Efficient Queries
Ensure that your database queries are optimized for pagination. Use indexing and avoid full table scans to improve performance, especially when dealing with large datasets.
4. Handle Changes in Data
Be prepared to handle changes in the dataset during pagination. Items may be added, removed, or updated between requests. Implement strategies to ensure consistency and handle edge cases gracefully.
5. Provide Pagination Controls
If your API is used by a front-end application, provide pagination controls to users. This might include "Next" and "Previous" buttons, page numbers, or infinite scrolling, depending on the user interface design.
6. Document Pagination Details
Document your pagination implementation clearly in the API documentation. Include information about the pagination strategy used, available parameters, and examples of requests and responses.
Implementing API Pagination: Example
Let’s look at an example of how to implement API pagination using cursor-based pagination. Assume you have an API for fetching a list of products.
1. Client Request: The client requests the first page of products.
2. API Response: The API returns the first page of products along with a cursor for the next page.
3. Subsequent Request: The client uses the provided cursor to fetch the next page.
4. Subsequent Response: The API returns the next page of products.
API pagination is a crucial technique for managing large datasets efficiently and enhancing user experience. By understanding and implementing pagination strategies effectively, you can optimize performance, reduce network traffic, and provide a seamless experience for users interacting with your API.
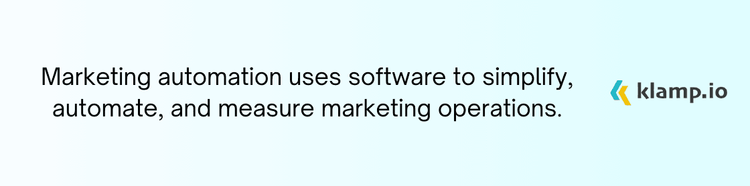