Python, with its versatile request's library, offers a powerful way to manage pagination and efficiently retrieve large sets of data. In this blog, we'll dive into how pagination works, why it’s essential, and how you can handle pagination using Python’s requests library.
What is Pagination?
APIs often limit the number of records returned in a single response due to performance reasons, bandwidth constraints, or to improve efficiency. This practice is known as pagination. Instead of receiving all the results at once, you're provided a subset, with added data accessible via "pages."
For example, when fetching records of user data, an API might limit responses to 100 records per page. If there are 1,000 total records, you’ll need to navigate through 10 pages to access all the data.
Pagination can come in various forms:
- Offset-based pagination: Uses an offset parameter to specify where the data starts.
- Cursor-based pagination: Uses a unique identifier (cursor) for the last retrieved record and fetches data after that cursor.
- Page number pagination: Specifies which page number you want to fetch.
Why Handle Pagination Efficiently?
Handling pagination is crucial for improving performance when fetching large datasets via APIs. By effectively managing pagination, you ensure that:
- Instead of bombarding a server with requests for huge datasets, you can request smaller chunks.
- Dealing with large datasets can overwhelm your system’s memory. By fetching smaller portions of data at a time, you avoid memory overload.
- Many APIs have rate limits, which restrict the number of requests you can make within a time. By handling pagination efficiently, you avoid breaching these limits.
Python Requests Library Guide
The requests library in Python is one of the most common packages for sending HTTP requests to interact with web services. Let’s first ensure the requests package is installed. If it’s not installed yet, you can use pip to install it:

Once installed, you can simply make GET requests to fetch data from an API. Pagination comes into play when the API limits how much data you can retrieve in a single request.
Example of Python Requests Pagination
Let’s now dive into an example where we handle pagination using Python requests. We’ll assume you’re working with an API that returns paginated data, such as a list of users. The API provides a page parameter to specify which page of results to retrieve and a per_page parameter to control the number of records per page.
Here’s a basic example of how you can use python requests pagination:
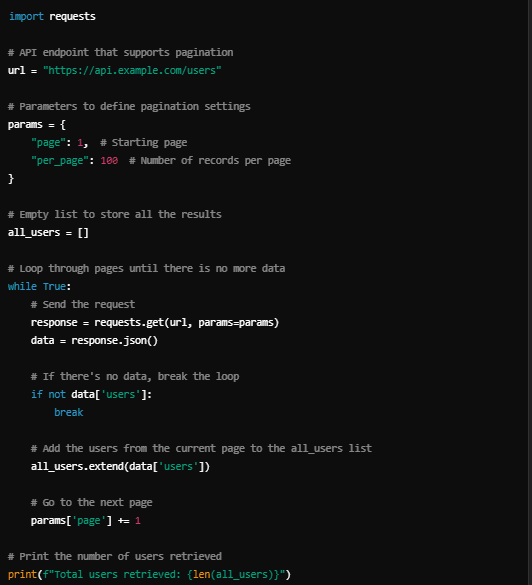
In the example above:
- We define the first page as 1 and set per_page to 100, meaning we’re fetching 100 users per page.
- We store all the retrieved users in the all_users list.
- A while loop ensures we keep requesting pages until no more data is returned.
- We increase the page number after each successful request to fetch the next set of users.
- This approach proves python requests pagination for a typical API that uses page numbers.
Dealing with Cursor-Based Pagination
Some APIs use a cursor-based pagination system, which is often more efficient than page-number pagination, particularly for large datasets that may change often. In cursor-based pagination, the API response will include a "cursor" or "next" token that you use to fetch the next batch of data.
Here’s how you might handle python requests pagination with cursor-based pagination:
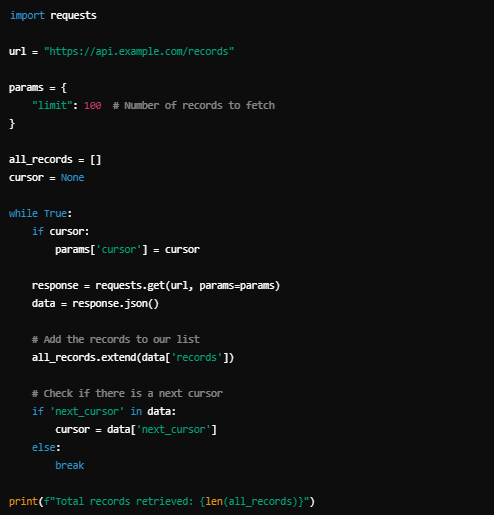
Tips for Python Requests Pagination Tips
While the above examples work well, there are some best practices to consider when implementing pagination in Python using the requests library.
Some APIs have rate limits, meaning you can only send a limited number of requests per minute. When handling python requests pagination, check the API’s documentation to see if it returns rate-limiting information in its headers. Use this information to add a delay between requests if necessary.
- Use Exponential Backoff for Retries: If the API server is temporarily unavailable, using exponential backoff can prevent overwhelming the server. This means that you increase the delay between retries with each attempt.
- Limit Memory Usage: If you are handling large amounts of data, consider saving it to disk or streaming it instead of holding everything in memory.
- Check for Edge Cases: Always account for potential edge cases such as empty pages, duplicate records, or missing data when implementing python requests pagination.
Pagination is a crucial concept when working with APIs that handle large datasets. By understanding and implementing python requests pagination, you ensure that your applications can retrieve data efficiently, avoid memory issues, and respect API rate limits. The Python requests library provides a powerful tool for managing pagination, whether through page numbers, cursors, or offsets.
