Pagination is a concept in web development and data handling, allowing users to navigate through large sets of data by dividing them into smaller, manageable pages. Whether you’re developing a web application, building an API, or simply managing large datasets, understanding pagination is essential. This blog delves into the intricacies of pagination in Python, offering practical examples and insights to help you implement effective pagination in your projects.
Pagination
Pagination is the process of dividing a large dataset into smaller chunks or "pages" and providing navigation controls to allow users to move between these pages. This technique is especially useful in scenarios were loading an entire dataset at once would be inefficient or overwhelming.
Why Use Pagination?
Performance: Loading all data at once can be slow and memory intensive. Pagination reduces the amount of data loaded at any given time, enhancing performance.
User Experience: Breaking down data into pages makes it easier for users to navigate and find the information they need.
Growth: Pagination helps manage large datasets by ensuring that only a subset of data is processed and displayed at any time.
Implementing Pagination in Python
Python offers several ways to implement pagination, depending on your use case and the type of application you are building. Let’s explore some common approaches.
1. Pagination in Web Applications
If you’re building a web application with frameworks like Flask or Django, pagination is often supported out-of-the-box or through extensions.
Flask Pagination
Flask, a lightweight web framework, doesn’t have built-in pagination support, but you can easily add it using the Flask-Pagination extension.
Django Pagination
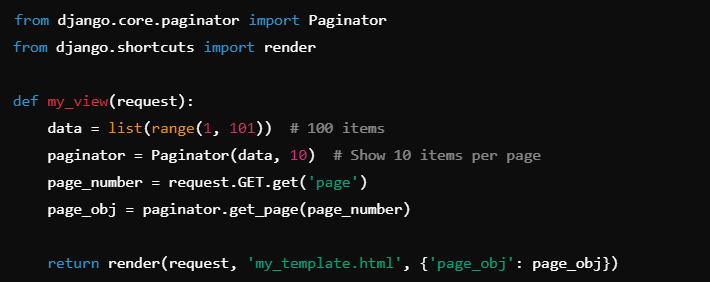
Here, Paginator handles the pagination logic, and ‘get_page’ retrieves the items for the current page. Django’s template system can then be used to display pagination controls.
2. Pagination in APIs
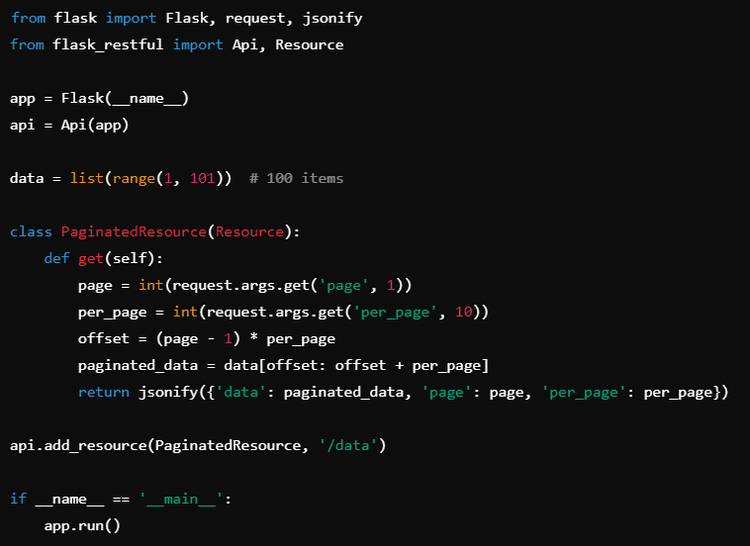
In this example, the API endpoint ‘/data’ supports pagination via ‘page’ and ‘per_page’ query parameters. The ‘get’ method calculates the offset and returns the appropriate subset of data.
3. Pagination in Data Analysis
For data analysis tasks, pagination can be used to process and analyze large datasets in chunks.
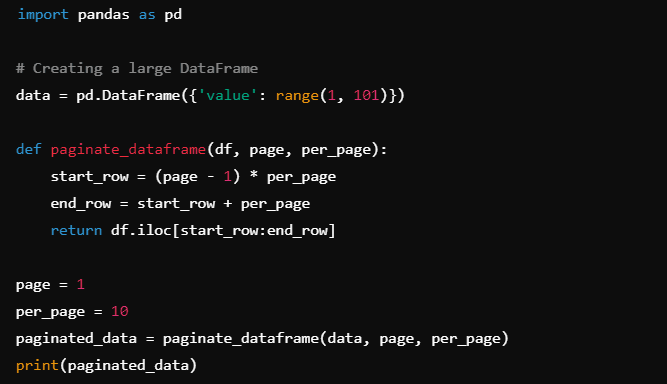
Here, the ‘paginate_dataframe’ function slices the ‘DataFrame’ to return only the rows for the specified page. This approach is useful for handling large datasets in chunks.
Tips for Pagination
Determine Page Size: Choose an appropriate number of items per page to balance performance and usability. Too many items can slow down page rendering, while too few can require excessive navigation.
Provide Navigation Controls: Ensure that users can easily navigate between pages using "Next", "Previous", "First", and "Last" controls.
Handle Edge Cases: Consider scenarios like empty pages or invalid page numbers. Ensure that your implementation gracefully handles such cases.
Optimize Performance: When dealing with large datasets, optimize your queries and data retrieval methods to avoid performance bottlenecks.
Pagination is a fundamental technique in managing large datasets, improving user experience, and improving performance. Whether you’re working on a web application, an API, or data analysis, Python provides versatile tools and libraries to implement effective pagination. By understanding and applying these concepts, you can build scalable and user-friendly applications that handle large volumes of data with ease.

"Discover Klamp Embed pricing and unlock affordable automation options with Klamp."