What is Pagination in REST API?
Pagination in REST APIs refers to the process of dividing a dataset into smaller pages that can be returned to the client in sequential requests. Instead of returning all the records at once (which can be slow and resource-intensive), the server provides only a part of the data per request. Clients can request the next page of data by specifying certain pagination parameters.
In essence, pagination improves performance, reduces response time, and allows the API to efficiently handle large datasets by providing partial responses.
Why Use Pagination in REST APIs?
Performance Optimization
Retrieving large datasets at once can cause delays, timeouts, or even service crashes. Pagination allows you to fetch data in smaller batches, reducing the risk of performance bottlenecks.
Improved User Experience
Clients receive data faster, and only the relevant part of the dataset is loaded into the UI or application.
Reduced Bandwidth Usage
With pagination, you only transfer the required data, lowering network traffic.
Lower Database Load
Retrieving fewer records at each request reduces the strain on the database, resulting in faster response times and lower infrastructure costs.
Common Pagination Techniques
There are several ways to implement pagination in REST APIs, each with its advantages and trade-offs. Let’s discuss the most popular methods:
1. Offset-Based Pagination
Offset-based pagination is one of the simplest and most used pagination methods. In this approach, the client specifies an offset (starting point) and a limit (number of records to fetch) as query parameters in the API request.
- Offset: Specifies the starting position in the dataset.
- Limit: Defines how many records to return.
For example, let’s say we have an API that returns a list of users. The request for paginated data might look like:

This request would return 10 users, starting from the 21st user (offset = 20).
Pros of Offset-Based Pagination:
- Easy to implement: This method is straightforward to implement in most databases.
- Predictable results: The client can request any page by specifying the offset.
Cons of Offset-Based Pagination:
- Slow with large datasets: As the offset increases, performance can degrade since the database still needs to scan through the earlier records.
- Inconsistent results: If the underlying dataset changes (e.g., records are added or deleted), the data on later pages may shift, leading to inconsistencies.
2. Cursor-Based Pagination
Cursor-based pagination (also known as keyset pagination) is a more efficient method for dealing with large datasets. Instead of using an offset, the client sends a cursor (a unique identifier) that points to the last item from the earlier page. The server returns the next set of results based on that cursor.
For example:

The cursor here is the last user from the earlier page, and the server will return the next 10 users after the one with this identifier.
Pros of Cursor-Based Pagination:
- More efficient: This method works faster for large datasets since it avoids the need to scan records up to the offset.
- Consistent results: Cursor-based pagination is less likely to suffer from inconsistencies if the dataset changes.
Cons of Cursor-Based Pagination:
- Complex to implement: Implementing cursors can be more complex than the offset-based approach, especially when dealing with composite keys.
- Less flexibility: It’s harder to jump to a specific page, as cursors are sequential and do not offer a direct way to skip pages.
3. Page Number-Based Pagination
In this method, pagination is based on specific page numbers rather than offsets or cursors. The client specifies a page number and the size (number of records) to retrieve.
Pros of Page Number-Based Pagination:
- Intuitive: Clients can easily understand and request specific pages.
- Easy to implement: This approach is simple to implement and works well for small datasets.
Cons of Page Number-Based Pagination:
- Deficient performance with large datasets: Like offset-based pagination, performance can degrade with large datasets.
- Inconsistent results: If the dataset changes, results across pages may shift.
How to Implement Pagination in REST API
Now that we understand the different pagination techniques, let’s dive into how to implement pagination in a REST API using popular technologies like Node.js with Express and Python with Flask.
Example 1: Implementing Offset-Based Pagination in Node.js with Express
First, let’s assume you have a ‘users’ table and want to implement pagination using an offset and limit.
Step 1: Set Up Express and Database

This request asks for the second page of users, with 10 users per page.
Pros of Page Number-Based Pagination:
- Intuitive: Clients can easily understand and request specific pages.
- Easy to implement: This approach is simple to implement and works well for small datasets.
Cons of Page Number-Based Pagination:
- Inferior performance with large datasets: Like offset-based pagination, performance can degrade with large datasets.
- Inconsistent results: If the dataset changes, results across pages may shift.
How to Implement Pagination in REST API
Now that we understand the different pagination techniques, let’s dive into how to implement pagination in a REST API using popular technologies like Node.js with Express and Python with Flask.
Example 1: Implementing Offset-Based Pagination in Node.js with Express
First, let’s assume you have a ‘users’ table and want to implement pagination using an offset and limit.
Step 1: Set Up Express and Database
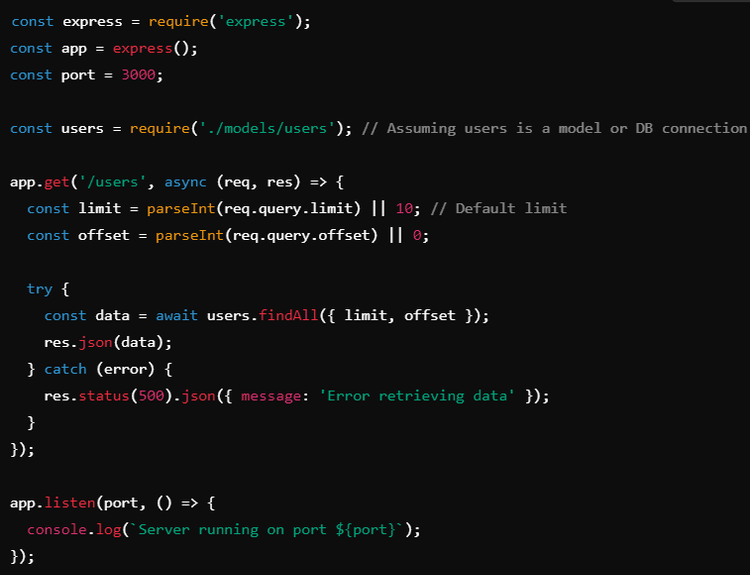
Here, we use limit and offset query parameters to control pagination. The default limit is set to 10, and the offset starts at 0.
Step 2: Test the API
You can test the API using a URL like:

This will return 5 users starting from the 11th user.
Example 2: Implementing Cursor-Based Pagination in Python with Flask
Let’s now look at how to implement cursor-based pagination using Flask and SQL Alchemy.
Step 1: Set Up Flask and Database
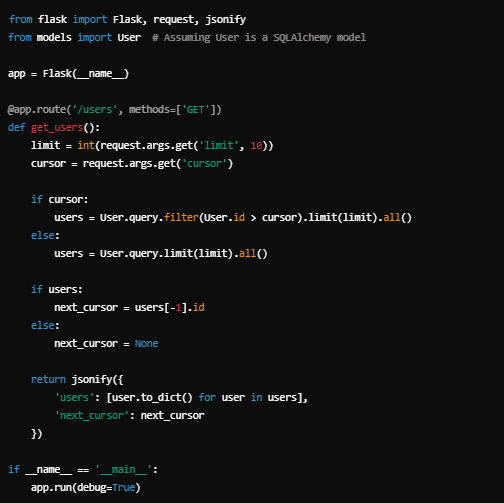
In this implementation, we use the cursor parameter to fetch records after a specific user ID.
Step 2: Test the API
You can test the cursor-based pagination with:

This will return to the next 10 users after the user with ID 5.
Best Practices for Implementing Pagination in REST APIs
When implementing pagination, here are a few best practices to consider:
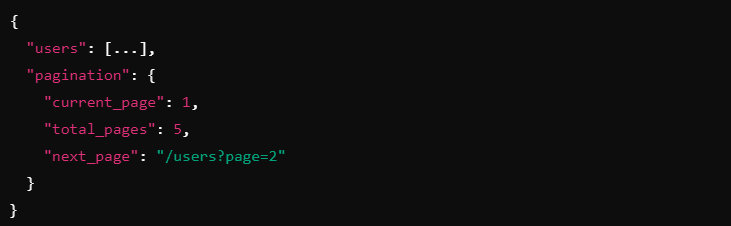
- Use Hypermedia Links: Including links to the next and earlier pages in the response body makes it easier for clients to navigate through paginated data.
- Limit the Maximum Page Size: To prevent clients from requesting an unreasonably considerable number of records, set a larger limit for the number of items that can be retrieved per page.
- Handle Edge Cases: Make sure to handle scenarios where no data is returned, such as when a client requests a page beyond the total number of pages.
- Document Pagination Parameters: Clearly document the pagination parameters (e.g., limit, offset, cursor) and their behavior in your API documentation.
Pagination is a critical feature in REST APIs, especially when dealing with large datasets. Whether you choose offset-based, cursor-based, or page-number-based pagination, implementing an efficient and scalable pagination strategy will improve the performance and usability of your API.
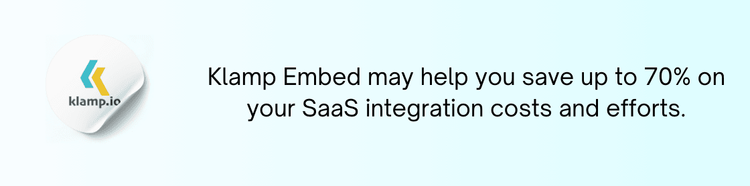